Memory-Safe-D-Spec
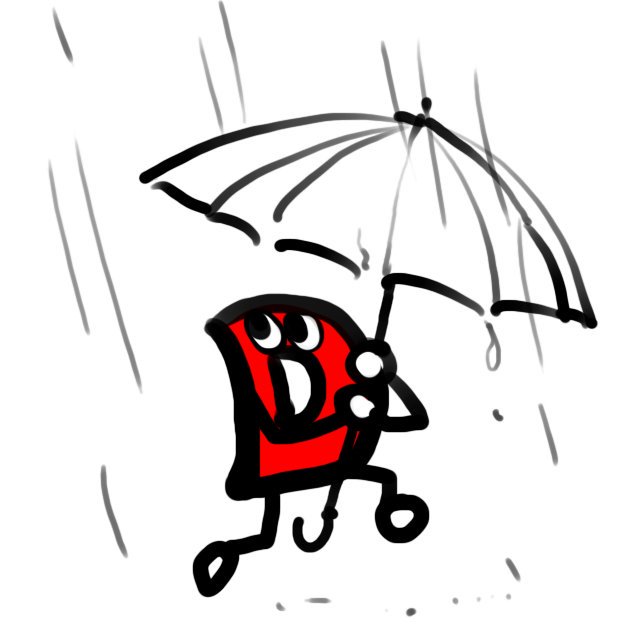
Memory Safety for a program is defined as it being impossible for the program to corrupt memory. Therefore, the safe subset of D consists only of programming language features that are guaranteed to never result in memory corruption. See this article for a rationale.
Memory-safe code cannot use certain language features, such as:
- Casts that break the type system.
- Modification of pointer values.
- Taking the address of a local variable or function parameter.
Usage
There are three categories of functions from the perspective of memory safety:
@system functions may perform any operation legal from the perspective of the language, including inherently memory unsafe operations such as pointer casts or pointer arithmetic. However, compile-time known memory-corrupting operations, such as indexing a static array out of bounds or returning a pointer to an expired stack frame, can still raise an error. @system functions may not be called directly from @safe functions.
@trusted functions must have a safe interface, as they can be called from @safe functions. Internally they have all the capabilities of @system functions. For this reason they should be very limited in the scope of their use. Typical uses of @trusted functions include wrapping system calls that take buffer pointer and length arguments separately so that @safe functions may call them with arrays.
@safe functions have a number of restrictions on what they may do and are intended to disallow operations that may cause memory corruption. See @safe functions.
The @safe attribute can be inferred when the compiler has the function body available, such as with templates.
Array bounds checks are necessary to enforce memory safety, so these are enabled (by default) for @safe code even in -release mode.
Scope and Return Parameters
The function parameter attributes return and scope are used to track what happens to low-level pointers passed to functions. Such pointers include: raw pointers, arrays, this, classes, ref parameters, delegate/lazy parameters, and aggregates containing a pointer.
The return ref attributes on a parameter indicate:
- A pointer derived from the parameter's address may be returned from the function (e.g. returning the parameter by reference).
- A pointer derived from the parameter's address may be stored in the first parameter of a function returning void, iff the first parameter is ref. The this reference is considered the first parameter of a method.
scope ensures that no references to the pointed-to object are retained, in global variables or pointers passed to the function (and recursively to other functions called in the function), as a result of calling the function. Variables in the function body and parameter list that are scope may have their allocations elided as a result.
The return scope attributes on a parameter indicate:
- A pointer derived from that parameter may be returned from the function.
- A pointer derived from that parameter may be stored in the first parameter of a function returning void, iff the first parameter is ref. The this reference is considered the first parameter of a method.
These attributes may appear after the formal parameter list of a method, in which case they apply to the this reference. For constructors, return applies to the (implicitly returned) this reference.
return or scope is ignored when applied to a type that is not a low-level pointer.
Note: Checks for scope parameters are currently enabled only for @safe code compiled with the -dip1000 command-line flag.
Limitations
Memory safety does not imply that code is portable, uses only sound programming practices, is free of byte order dependencies, or other bugs. It is focussed only on eliminating memory corruption possibilities.